In this Article, we will learn different ways of python dictionary updates.
Python Dictionary Update:
Let’s create a dictionary myDict as below having name and marks for students.
myDict={"Rohan":34,"Sonu":35,"Ram":45}
myDict
Output: {'Rohan': 34, 'Sonu': 35, 'Ram': 45}
Suppose we need to add marks for Sohan as 46. You Can use dictionary[‘key’] = value, to add or update a value for a python dictionary key. In this case, Sohan is key and 46 is value.
myDict["Sohan"]=46
myDict
Output:{'Rohan': 34, 'Sonu': 35, 'Ram': 45, 'Sohan': 46}
Python Dictionary Update Method:
Suppose we need to add marks for another student savik as 41. We can also use a builtin python dictionary method ‘update()‘ to add new key: value as shown below.
myDict.update({"savik":41})
myDict
Output : {'Rohan': 34, 'Sonu': 35, 'Ram': 45, 'Sohan': 46, 'savik': 41}
Python Dictionary Update If Key Exists.
So Far, we have updated the Dictionary by adding a new key and value entry. We can also update any Existing python dictionary keys. Suppose we incorrectly updated the marks for Ram. It’s not 45 but it’s 25. You can update the key ‘Ram’ as below:
myDict['Ram']=25
myDict
Output : {'Rohan': 34, 'Sonu': 35, 'Ram': 25, 'Sohan': 46, 'savik': 41}
If you try to add another entry for same key ‘Ram’ , it should not add new entry for same key, rather it will update the same key with updated value. that is the python dictionary Keys should be unique.
myDict.update({'Ram':35})
myDict
Output: {'Rohan': 34, 'Sonu': 35, 'Ram': 35, 'Sohan': 46, 'savik': 41}
Python Dictionary Update Using Two Python Lists:
Suppose we have two list one with student’s name and another with student’s marks. We wanted to create a dictionary from the two python lists.
#Let's see the two lists
name=['Rohan','Sonu','Ram','Sohan','savik']
marks=[34,35,35,46,41]
we can loop through both the list and pick key from name list and value from marks list.
myNewDict={}
for i,j in name,marks :
myNewDict[i]=j
# We hit an error when we try to run loop with two list this way
ValueError Traceback (most recent call last)
<ipython-input-20-d96841d3526f> in <cell line: 2>()
1 myNewDict={}
----> 2 for i,j in name,marks:
3 myNewDict[i]=j
ValueError: too many values to unpack (expected 2)
# To fix this issue , we need to use zip function .
myNewDict={}
for i,j in zip(name,marks):
myNewDict[i]=j
myNewDict
Output : {'Rohan': 34, 'Sonu': 35, 'Ram': 35, 'Sohan': 46, 'savik': 41}
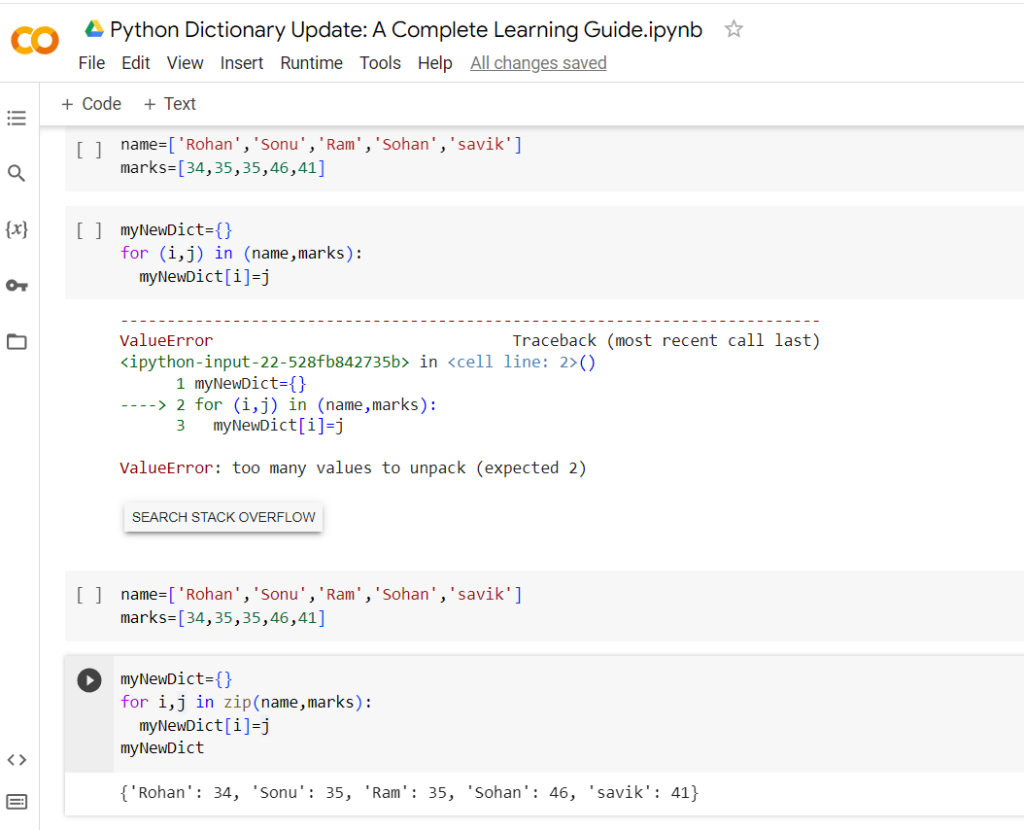
Want to Learn Python from our Expert . Check this out .
Don’t trust us , See if you like learnpython.com