In this article, we will learn the fundamentals of creating Python Function by our own. Let’s take an example.
Python function Problem Statement:
Create a function which will add all single digit numbers from a given n digit number.
For Example ,if n digit number is like 1234567 , then the function should return 1+2+3+4+5+6+7 =28.
To understand the logic, consider running a loop to iterate through all the single digit numbers of 1234567 and keep adding them. However, a critical issue arises: the ‘int’ object is not iterable. This means you can’t iterate through integer value. If you try to run Below Code:
for i in 123456:
print(i)
You get below output :
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[4], line 1
----> 1 for i in 123456:
2 print(i)
TypeError: 'int' object is not iterable
To ensure iterability, you must convert it into a string. This will result in individual digits like 1, 2, 3, and so forth, each represented as a string rather than a numeric value. To perform addition, you’ll then need to convert them back to integers before applying the summation process. If you try to run Below Code:
for i in str(123456):
print(i)
You get below output :
1
2
3
4
5
6
Actual Code:
Keeping this in mind let’s write the function.
def SumNumber(n):
#Initiate a variable 'sm' as 0, where you keep on adding all single digit's numbers
sm=0
#iterate through the number given and to do that convert that number into string using str() function.
for i in str(n):
#For each character, convert them back to an integer and add into sm
sm=sm+int(i)
#Once the loop is complete return 'sm' which must have stored the sum of all single digit numbers in given number.
return sm
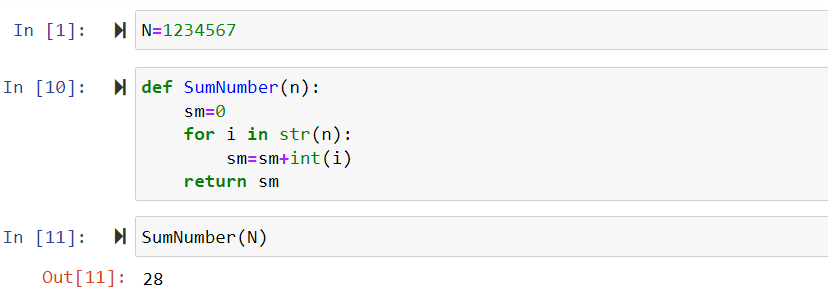
If you have any doubt please comment below or Contact us and we will be happy to help .
To learn Python check out this .
Check out our courses :here
Right here is the perfect web site for anyone who wishes
to understand this topic. You understand a whole lot its almost hard to argue with you (not that I really would want to…HaHa).
You certainly put a fresh spin on a topic that
has been written about for decades. Excellent stuff, just great!
Thanks really appreciate that.