In this Article, we will find the length of python list. Understanding the list length is crucial when implementing complex program logic. While it’s possible to determine it through a custom program , Python offers a built-in function for this common task.
Program to find python list length
We can iterate through the list and continuously increment a counter variable to determine its length.
mylist=[23,45,12,87,38]
counter=0
for i in mylist:
counter=counter+1
print(counter)
Output: 5
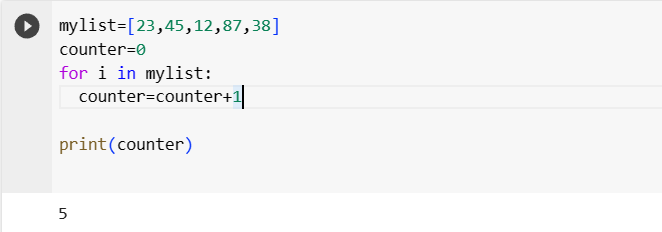
python list length using len() function
You don’t have to create a custom program for list length determination. Python offers a built-in len
() function for precisely that purpose. The primary method for determining the length of a Python list is by utilizing the built-in len() function. In Python, len() is a function designed to accept a single argument and provide the count of elements or items within that argument. This argument can be any type of iterable, including dictionaries, tuples, lists, and even custom objects.
mylist=[23,45,12,87,38]
counter=len(mylist)
print(counter)
Output : 5
Refer the code : here
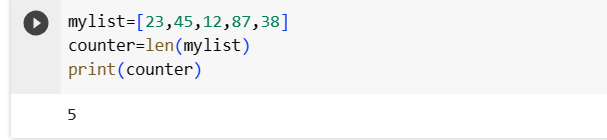
Using Custom Function over len()
When You have to find a list length but include even numbers in the list . In this case , len() function can’t be used and we have to write a custom program to find length of list include only even number from the list. In other words the problem statement will be find the list containing the maximum number of even numbers. To do this you need a function to find number of even number in the list as below .
mylist=[23,45,12,87,38]
counter=0
def lenEven(lst,counter=0):
for i in mylist:
if i%2==0:
counter=counter+1
else:
continue
return counter
lenEven(mylist)
Output: 2
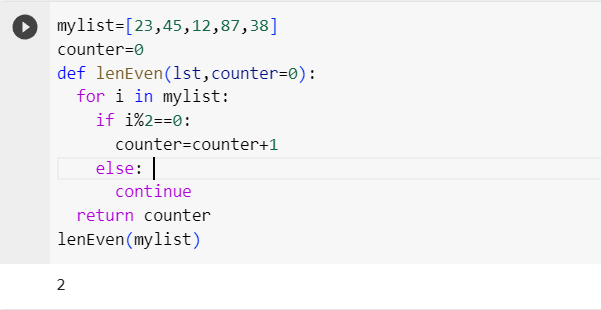
Check out our courses :here
If you have any doubt please comment below or Contact us and we will be happy to help .
To learn Python check out this .